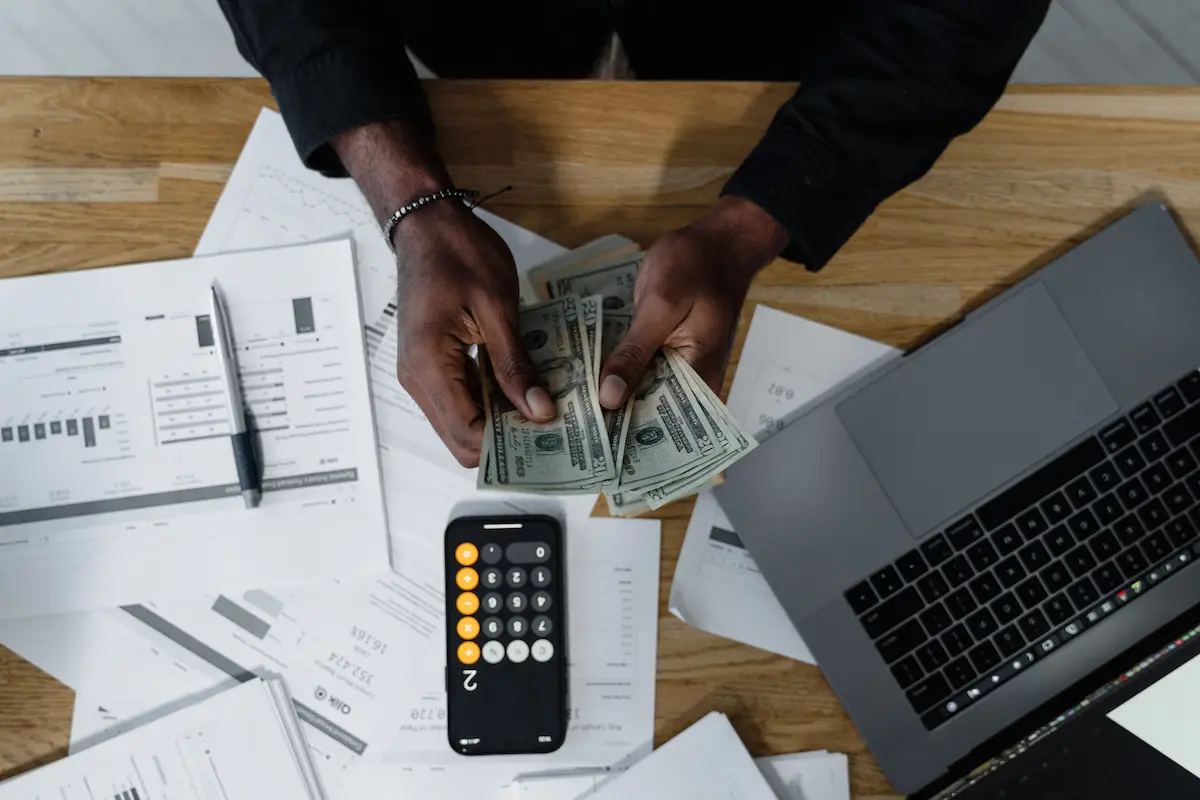
React Native has taken the mobile development world by storm, offering a powerful and efficient way to build cross-platform applications. With its ability to use JavaScript to create native mobile apps for both iOS and Android, React Native has become a popular choice for developers looking to streamline their development process and reach a wider audience.
In this comprehensive guide, we will dive into the world of React Native and explore everything you need to know to get started with this powerful framework. From understanding its architecture to mastering performance optimization techniques, we will cover it all. So let’s get started on our journey to becoming a React Native pro!
Building Mobile Apps with React Native: Step-by-Step Tutorial
Before we delve into the technical details of React Native, let’s first understand how to actually build a mobile app using this framework. The following step-by-step tutorial will guide you through the process of creating a simple “Hello World” app with React Native.
Setting Up Your Development Environment
The first step in building a React Native app is to set up your development environment. You will need to have Node.js installed on your system, as well as a code editor of your choice. Additionally, you will need to install the React Native command line interface (CLI) using the following command:
npm install -g react-native-cli
Creating a New Project
Once your development environment is set up, you can create a new React Native project using the following command:
react-native init HelloWorld
This will create a new folder named “HelloWorld” containing all the necessary files and folders for your project.
Running the App
To run your app on a simulator or device, navigate to the project directory and run the following command:
react-native run-ios
This will launch the app on an iOS simulator. To run it on an Android emulator, use the following command:
react-native run-android
Congratulations! You have successfully created and launched your first React Native app.
Unveiling the Architecture of React Native: A Deep Dive
Now that you have a basic understanding of how to build a React Native app, let’s take a closer look at its architecture. React Native follows a hybrid approach, combining the best of both native and web development. This allows for faster development and better performance compared to other cross-platform frameworks.
React Native Components
At the core of React Native are components, which are reusable building blocks for creating user interfaces. These components are written in JavaScript and are then translated into native code by React Native. This allows for a consistent look and feel across different platforms while still maintaining the performance benefits of native code.
Virtual DOM
React Native also uses a virtual DOM (Document Object Model) to efficiently update the UI. The virtual DOM is a representation of the actual DOM and allows React Native to make changes to the UI without directly manipulating the DOM. This results in faster rendering and improved performance.
Bridge
The bridge is another important component of React Native’s architecture. It acts as a communication channel between the JavaScript code and the native code. Whenever a component needs to interact with the native code, it sends a message through the bridge, which then translates it into the appropriate native code.
React Native Performance Optimization Techniques for Seamless Apps
One of the major advantages of using React Native is its ability to deliver high-performance mobile apps. However, there are certain techniques that can be used to further optimize the performance of your React Native apps.
Use FlatList Instead of ScrollView
When displaying a large list of data, it is recommended to use the FlatList component instead of ScrollView. FlatList is optimized for rendering large lists and only renders the items that are currently visible on the screen. This results in improved performance and reduced memory usage.
Use Native Code for CPU-Intensive Tasks
While React Native allows you to write most of your code in JavaScript, there may be certain tasks that require more processing power. In such cases, it is recommended to use native code instead of JavaScript. This can be achieved by creating a native module and calling it from your JavaScript code.
Use Pure Components
Pure components are a type of React component that only re-renders when its props or state change. This can significantly improve the performance of your app by reducing unnecessary re-renders. To create a pure component, simply extend the PureComponent class instead of the regular Component class.
Mastering Cross-Platform Development with React Native: Strategies and Best Practices
One of the main reasons for choosing React Native is its ability to build cross-platform apps. However, there are certain strategies and best practices that can help you make the most out of this feature.
Keep Platform-Specific Code to a Minimum
While React Native allows you to share a majority of your code between different platforms, there may be certain cases where you need to write platform-specific code. It is important to keep this code to a minimum and only use it when necessary. This will ensure that your app remains consistent across different platforms.
Test on Real Devices
It is important to test your app on real devices rather than just simulators. This will give you a better understanding of how your app performs on different devices and help you identify any potential issues. Additionally, you can also use tools like Expo to easily test your app on multiple devices at once.
Follow Platform Guidelines
Each platform has its own set of design guidelines and best practices. It is important to follow these guidelines when building your app to ensure a seamless user experience. For example, iOS and Android have different navigation patterns and it is important to follow them accordingly.
Exploring the React Native Ecosystem: Essential Tools and Libraries
React Native has a thriving ecosystem with a wide range of tools and libraries that can help you build better apps. Here are some essential tools and libraries that every React Native developer should be familiar with.
Expo
Expo is a set of tools and services that make it easier to develop, build, and deploy React Native apps. It provides a development environment, an app store for sharing your projects, and various APIs for accessing device features like camera, location, and push notifications.
Redux
Redux is a popular state management library that can be used with React Native to manage complex application states. It allows you to store all your app’s data in a single global state object, making it easier to access and modify data from different components.
React Navigation
React Navigation is a routing and navigation library for React Native. It provides a simple and customizable way to handle navigation between screens in your app. It also supports deep linking, which allows users to navigate directly to a specific screen within your app.
Debugging and Troubleshooting React Native Applications: Common Issues and Solutions
As with any software development, there may be times when you encounter issues or bugs while working with React Native. Here are some common issues and solutions that can help you troubleshoot your React Native apps.
Red Screen of Death (RSOD)
The Red Screen of Death is a common issue in React Native where the app crashes and displays a red error screen. This usually happens when there is an error in your code or when a native module fails to load. To fix this, check your code for any errors and try reinstalling the app on your device.
Slow Performance
If your app is running slowly, there are a few things you can do to improve its performance. First, make sure you are using FlatList instead of ScrollView for large lists. You can also use the Performance Monitor tool in React Native Debugger to identify any bottlenecks in your code.
Debugging with React Native Debugger
React Native Debugger is a powerful tool that allows you to debug your React Native apps using Chrome Developer Tools. It provides a more comprehensive debugging experience compared to the default debugger and can help you identify and fix issues more efficiently.
Building Real-World React Native Applications: Case Studies and Examples
To truly understand the power and potential of React Native, let’s take a look at some real-world applications built with this framework.
Facebook Ads Manager
Facebook Ads Manager is a mobile app used by businesses to manage their Facebook ad campaigns. The app was originally built using native code but was later rewritten in React Native. This resulted in a significant reduction in development time and improved performance.
Instagram, one of the most popular social media platforms, also uses React Native for its mobile app. The app has a consistent and seamless user experience across different platforms, thanks to React Native’s cross-platform capabilities.
Tesla
Tesla’s mobile app, used to control and monitor their electric cars, is also built with React Native. This allowed Tesla to quickly release updates and new features for both iOS and Android without having to maintain separate codebases.
The Future of React Native: Upcoming Trends and Innovations
As React Native continues to evolve, there are several upcoming trends and innovations that we can expect to see in the near future.
Fabric
Fabric is a new architecture for React Native that aims to improve performance and make it easier to integrate with existing native code. It is currently in the experimental stage and is expected to be released soon.
Hermes
Hermes is a new JavaScript engine developed by Facebook specifically for React Native. It is designed to improve the startup time and memory usage of React Native apps, making them even faster and more efficient.
React Native for Web
React Native for Web is a project that aims to bring the power of React Native to the web. This will allow developers to build cross-platform apps for mobile, web, and desktop using a single codebase.
React Native vs. Other Cross-Platform Frameworks: A Comparative Analysis
While React Native is undoubtedly a popular choice for cross-platform development, there are other frameworks available as well. Let’s take a look at how React Native compares to some of its competitors.
React Native vs. Flutter
Flutter is a cross-platform framework developed by Google. While both React Native and Flutter use a hybrid approach, Flutter uses its own rendering engine instead of relying on native components. This results in better performance but also limits the ability to customize the UI.
React Native vs. Xamarin
Xamarin is another popular cross-platform framework that uses C
instead of JavaScript. While it offers better performance compared to React Native, it requires developers to have knowledge of C# and the .NET framework.
React Native vs. Ionic
Ionic is a popular hybrid app development framework that uses web technologies like HTML, CSS, and JavaScript. While it allows for faster development, it may not offer the same level of performance as React Native due to its reliance on web technologies.
Conclusion
In this comprehensive guide, we have covered everything you need to know to get started with React Native. From understanding its architecture to mastering performance optimization techniques, we have explored all aspects of this powerful framework. We have also looked at real-world examples and upcoming trends in the world of React Native.
With its ability to create high-performance cross-platform apps, React Native has become a go-to choice for many developers. So why not give it a try and see for yourself the endless possibilities it offers for building seamless mobile applications. Happy coding!